Table Of Content
Test-Driven Development (TDD) is a methodology that emphasizes writing tests before coding to build high-quality software. By focusing on testing small units of functionality, TDD ensures that code is thoroughly tested, leading to fewer bugs and higher overall quality.
This approach aligns well with Agile and XP practices, promoting iterative development, continuous feedback, and simple design. TDD not only enhances the robustness and maintainability of the software but also fosters a disciplined development process that can adapt to changing requirements and deliver reliable, customer-focused solutions.
Core Principles of TDD
- Writing Tests First: In TDD, tests are written for small units of functionality (unit tests) or features, and testing is repeated until all criteria are validated. This ensures that requirements are converted to test cases and that testing is comprehensive before the code is fully developed.
- Focus on Testability: TDD is highly effective because it builds the application’s functionality based on its testability. Units (or modules) are kept small for easier understanding and to ensure that the acceptance criteria are met.
The TDD Cycle
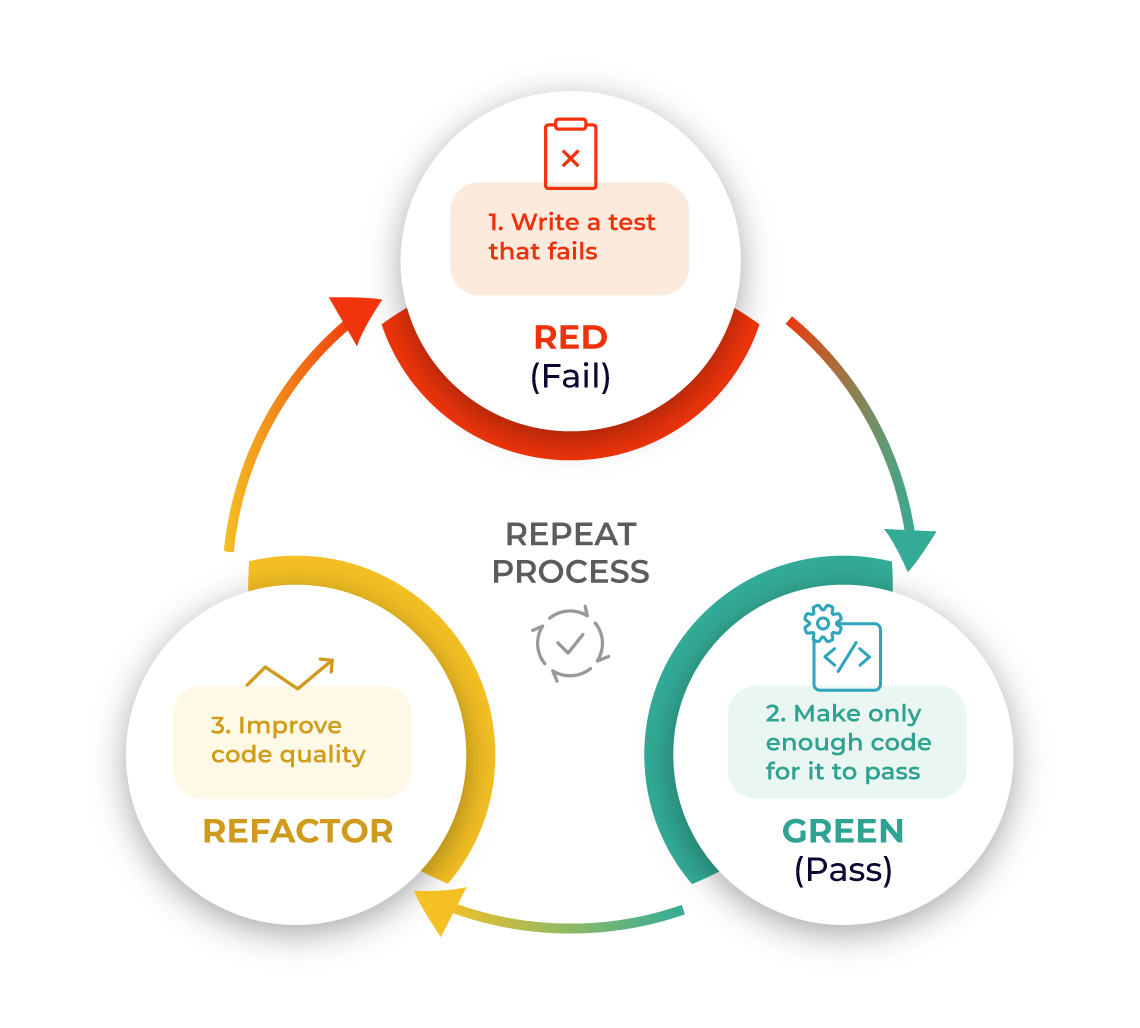
- Red Phase: Write a test for the next piece of functionality you want to add. This test should focus on a single aspect of the behavior you want to implement. Execute the test suite to ensure the new test fails. The test should fail because the functionality has not yet been implemented.
addition_test(){
Assert.assertTrue(add(2,3) == 5));
}
- Green Phase: Write the minimal code necessary to make the failing test pass. The focus here is on getting the test to pass quickly rather than writing perfect code. Execute the test suite again to see if the new test passes. If it passes, it indicates that the code works for the specific case tested.
public int add(int a, int b){
return a + b;
}
- Refactor Phase: Review and improve the code once the test passes. This may involve cleaning up the implementation, removing duplication, improving readability, or optimizing performance. Ensure that all tests still pass after refactoring. This confirms that the refactoring has yet to introduce any new issues.
Benefits of TDD
- Improved Code Quality: TDD ensures that code is thoroughly tested as it is written, leading to fewer bugs and higher overall quality. Writing tests first forces developers to consider edge cases and requirements upfront, reducing the likelihood of defects later.
- Better Design and Architecture: By focusing on writing tests first, developers are encouraged to think about the design and interfaces of their code before implementation. This often leads to more modular, loosely coupled, and easier-to-maintain code.
- Faster Debugging: When tests are written first, any new code that causes a test to fail can be quickly identified and fixed. This reduces the time spent debugging and chasing down issues, as the tests provide immediate feedback on the correctness of the code.
- Increased Confidence in Code Changes: A comprehensive suite of tests allows developers to make changes and refactor code confidently. If all tests pass after a change, it indicates that the change did not introduce any new bugs, which is especially valuable in large and complex codebases.
- Documentation: Tests serve as living documentation for the codebase. They describe how the code is supposed to behave, making it easier for new team members to understand the system and for existing members to recall the intended behavior of different parts of the system.
TDD in Agile and XP Development
- Popularity in Agile: Test-driven development techniques have become very popular in agile development. They encourage simple design and test suites that inspire confidence.
- Alignment with XP: TDD is a core practice of XP (Extreme Programming), emphasizing simplicity, feedback, and incremental development. This alignment supports robust software quality and maintainability.
- Testing Specifications: TDD is a process of testing the specification before writing the code and implementing the business logic, ensuring software quality and preventing code issues before integration with other components.
- Impact on Software Quality: TDD boosts the quality of system components, speeds up time to market, shortens development cycles, and supports new requirements.
Challenges of TDD
- Initial Learning Curve: Developers new to TDD may find it challenging to write tests before code and to think in small, incremental steps. Providing adequate training and support can help overcome this initial hurdle.
- Time-Consuming: Writing tests adds to the development time. Although this can initially slow development, it reduces debugging and maintenance efforts, saving time in the long run.
- Neglecting Refactoring: One of the most common mistakes is failing to refactor the code after making the tests pass. This can lead to functional code that is difficult to read, maintain, or extend. Refactoring is crucial in the TDD cycle to keep the codebase clean and manageable.
- Overly Complex Tests: Writing overly complex or tightly coupled tests can make maintenance easier. Tests should be simple, focused, and isolated. Complex test setups can indicate that the code being tested is too complex and may need refactoring.
- Ignoring Integration and System Testing: TDD primarily focuses on unit tests, which are essential but insufficient for ensuring overall system quality. Teams should also include integration and system tests to cover interactions between different modules and external systems.
- Test Duplication: Duplication in tests can lead to maintenance overhead. Ensure that tests are not redundant and that each test case adds unique value. Regularly review and clean up the test suite to avoid unnecessary duplication.
- Inadequate Test Coverage: While TDD promotes writing tests first, it’s possible to miss edge cases or scenarios. Ensuring comprehensive test coverage is essential to fully leveraging TDD’s benefits. Use code coverage tools to identify untested parts of the codebase.
- Complex Test Scenarios: Writing tests for complex scenarios or legacy systems can be difficult and may require significant setup. It is important to invest the time needed to write these tests to ensure comprehensive coverage.
- Resistance to Change: Teams may resist adopting TDD due to the initial learning curve and the perception that it slows development. It’s important to provide training and demonstrate the long-term benefits of TDD to overcome this resistance.
- Misunderstanding the TDD Process: Some teams may misunderstand TDD as merely writing tests before code. TDD is about driving the design and implementation through tests. It’s crucial to internalize and use the “Red-Green-Refactor” cycle effectively.
Test-Driven Development Tools and Frameworks
xUnit frameworks are a family of unit testing frameworks that provide tools and libraries to help developers write and execute tests for their code. The “x” in xUnit is a placeholder for various programming languages, indicating multiple implementations of the same basic testing concepts tailored to different programming environments. These frameworks are widely used in Test-Driven Development (TDD) practices and other testing methodologies.
Key Features of xUnit Frameworks
1. Test Case Management:
- xUnit frameworks allow developers to define test cases, which are individual tests that check specific aspects of the code’s functionality.
- Test cases are typically organized into test suites, which are collections of related tests.
2. Assertions:
- xUnit frameworks provide a set of assertion methods that developers use to verify the expected outcomes of their tests.
- Common assertions include checking for equality, checking for null or not null, checking for exceptions, and more.
3. Test Runners:
- xUnit frameworks include test runners that automate the execution of tests.
- Test runners provide feedback on which tests passed, which failed, and why failures occurred.
4. Fixtures and Setup/Teardown Methods:
- xUnit frameworks support setup and teardown methods that run before and after each test case or test suite.
- These methods help set up the test environment and clean up after tests run, ensuring that tests do not interfere.
5. Mocking and Stubbing:
- Many xUnit frameworks integrate with mocking libraries, allowing developers to create mock objects and stubs for testing purposes.
- This helps isolate the unit of code being tested and simulate dependencies.
Popular xUnit Frameworks
JUnit (Java)
- Description: JUnit is a widely used testing framework for Java applications. It annotates test methods, setup/teardown methods, and test suites.
- Features:
- Annotations like @Test, @Before, @After, @BeforeClass, and @AfterClass.
- Assertions like assertEquals, assertTrue, assertFalse, assertNull, and assertNotNull.
- Integration with IDEs and build tools like Maven and Gradle.
NUnit (C#)
- Description: NUnit is a popular testing framework for .NET applications. It offers a rich set of features for writing and running tests.
- Features:
- Attributes like [Test], [SetUp], [TearDown], [OneTimeSetUp], and [OneTimeTearDown].
- Assertions like Assert.AreEqual, Assert.IsTrue, Assert.IsFalse, Assert.IsNull, and Assert.IsNotNull.
- Support for parameterized tests and data-driven testing.
PyTest (Python)
- Description: PyTest is a robust testing framework for Python. It is known for its simplicity and powerful features.
- Features:
- Simple syntax for writing tests using functions.
- Fixtures for setup and teardown logic.
- Support for plugins and extensions.
- Assertions using Python’s built-in assert statement.
RSpec (Ruby)
- Description: RSpec is a testing framework for Ruby, commonly used for behavior-driven development (BDD).
- Features:
- Domain-specific language (DSL) for writing tests in a readable format.
- Describes test cases using describe and it blocks.
- Built-in matches for assertions, such as expect(x).to eq(y) and expect { … }.to raise_error.
Jest (JavaScript)
- Description: Jest is a JavaScript testing framework maintained by Facebook that is commonly used with React applications.
- Features:
- Zero-configuration setup and easy integration with projects.
- Built-in matchers like expect(value).toBe(expected) and expect(value).toEqual(expected).
- Snapshot testing for React components.
- Mocking capabilities for functions and modules.
Benefits of xUnit Frameworks
- Standardization: xUnit frameworks provide a standardized way to write and organize tests, making it easier for teams to maintain and understand test code.
- Automation: These frameworks support automated test execution, which helps in continuous integration and continuous deployment (CI/CD) pipelines.
- Isolation: By supporting setup and teardown methods, xUnit frameworks ensure that tests are isolated and do not interfere with each other, leading to more reliable test results.
- Feedback: xUnit frameworks provide immediate feedback on test outcomes, helping developers quickly identify and fix issues.
By using xUnit frameworks, developers can ensure their code is thoroughly tested, leading to higher-quality software and more robust applications.
Integrating TDD into the Development Process:
- Development Process:
- Adopt the TDD Cycle: Ensure all developers are trained and understand the “Red-Green-Refactor” cycle. Encourage writing tests before coding new features or fixing bugs.
- Pair Programming and Code Reviews: Utilize pair programming to facilitate knowledge sharing and ensure adherence to TDD practices. Conduct regular code reviews to ensure that tests are written first and to maintain code quality.
- Small, Incremental Changes: Break down features and tasks into small, manageable pieces that can be developed and tested incrementally. Write tests for each small piece of functionality to ensure comprehensive coverage.
- Testing Process:
- Automated Testing Frameworks: Write and run tests using automated testing frameworks like JUnit (Java), NUnit (C#), RSpec (Ruby), PyTest (Python), or Jest (JavaScript). Ensure tests are part of the build process and run automatically whenever code is committed.
- Continuous Integration (CI): Set up a CI server (e.g., Jenkins, Travis CI, CircleCI) to automatically run tests whenever changes are pushed to the repository. Configure the pipeline to provide the development team with immediate feedback on test results.
- Code Coverage Tools: Use code coverage tools (e.g., JaCoCo, Istanbul, Coveralls) to measure and track test coverage. Aim for high code coverage but ensure that the focus remains on meaningful tests rather than just increasing the coverage percentage.
- Deployment Process:
- Continuous Deployment (CD): Integrate Continuous Integration (CI) with continuous deployment pipelines to automate the deployment process and run all the TDD-produced tests as part of those pipelines. Ensure that only code that passes all tests in the CI pipeline is deployed to production or staging environments.
- Staging Environment: Deploy to a staging environment before production to catch any integration issues that unit tests may not cover. Perform additional testing, such as user acceptance testing (UAT) and performance testing, in the staging environment.
- Rollback Strategies: Implement rollback strategies to quickly revert to a previous stable version if a deployment introduces issues. Ensure that the deployment process is automated and can handle rollbacks smoothly.
- Team Culture and Practices:
- Training and Education: Provide regular training sessions and workshops on TDD and related practices. Encourage continuous learning and sharing of best practices within the team.
- Encourage Collaboration: Foster a collaborative environment where developers, testers, and operations teams work closely. Promote open communication and regular feedback to improve the TDD process continuously.
- Metrics and Monitoring: Track metrics such as test pass/fail rates, code coverage, and deployment success rates to measure the effectiveness of TDD. Use these metrics to identify areas for improvement and to celebrate successes.
Workflow Integration
Let’s now examine an example of performing TDD in a complex system from its beginning to its deployment in production, maintenance, and eventual error corrections.
- Planning:
- Define user stories and acceptance criteria.
- Write high-level acceptance tests for each user story.
- Development:
- Follow each user story’s TDD cycle (Red-Green-Refactor) to implement the required functionality.
- Write unit tests first, implement the code, and then refactor.
- Testing:
- Run automated tests locally before committing code, such as those created before the code was developed.
- Push changes to the version control system, triggering the CI pipeline.
- CI pipeline runs all tests and provides feedback.
- Deployment:
- Upon passing all tests, the code is deployed to the staging environment for further testing.
- After successful testing in staging, deploy the code to production.
- Monitor production deployments and be ready to roll back if issues arise.
- Retrospective and Improvement:
- Regular retrospectives should be conducted to review the TDD process and identify areas for improvement.
- Implement changes based on feedback and continue to refine the process.
Krasamo App Development Services
TDD is an approach any development team can implement in their application lifecycle. By adopting TDD, agile teams will experience fewer bugs and gain a deeper understanding of the purpose behind their code even before it is written. Numerous tools and languages support this methodology, making it accessible to any development team. The key is to start the process and continuously refine it as you go.
Ready to elevate your software development process with TDD and agile testing practices? Contact a Krasamo engineer today to discuss how we can help you implement these methodologies and achieve higher quality, more maintainable software.
0 Comments